flask
https://www.udemy.com/course/python-api-programming-with-fastapi-and-flask/learn/lecture/
micro web framework
개발환경세팅 (web-apis-with-python)
Fork
https://github.com/CloudBytesDotdev/web-apis-with-python
clone
$ git clone https://github.com/sixxchung/web-apis-with-python
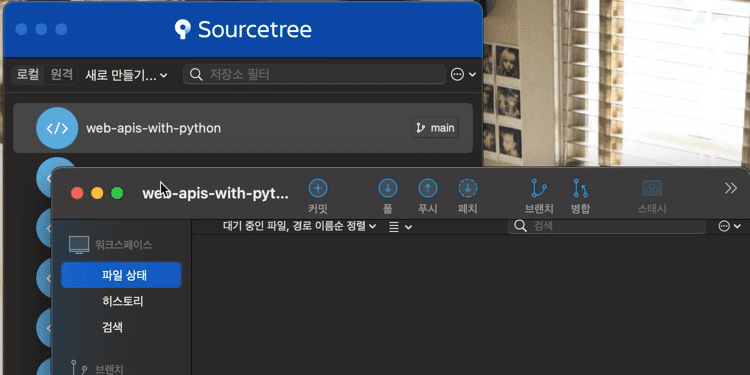
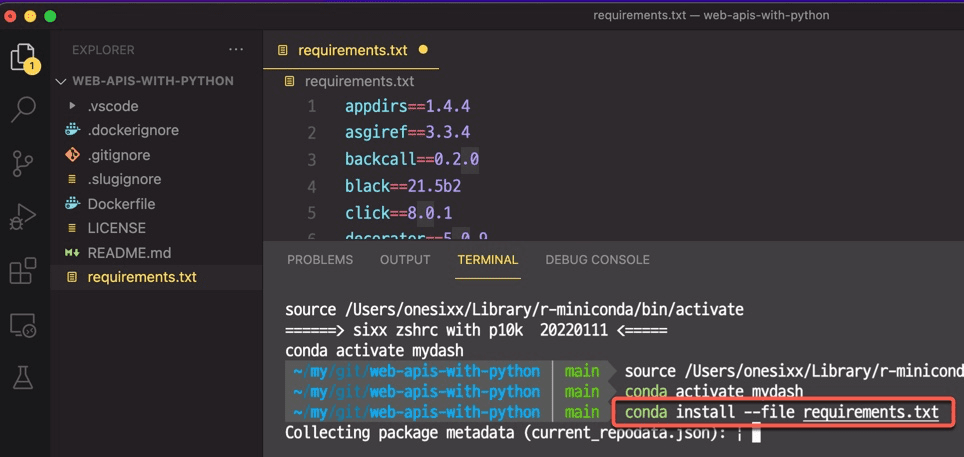
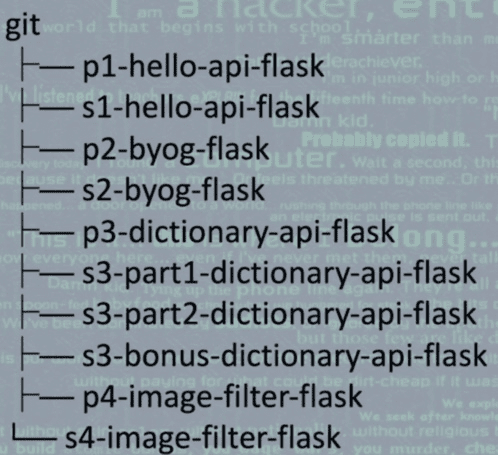
p1-hello-api-flask
# 임시로 변경사항 저장 $ git stash #$ git stash pop $ git checkout p1-hello-api-flask #$ pip3 install -r requirements.txt #$ conda update --all $ conda install --file requirements.txt $ flask run $ curl http://127.0.0.1:5000/greet $ curl -i -X GET http://127.0.0.1:5000/greet # --include 출력에 프로토콜 반응헤더 포함 # 사이트의 Header와 바디정보를 함께 가져오기 # -X : 사용할 (요청) 방식 메소드 선택하기
argument 와 에러처리
from flask import Flask, jsonify, request # Intitialise the app app = Flask(__name__) @app.get("/") def redirect_root(): return redirect("http://127.0.0.1:5000/greet") #return ("Hello world") # Define what the app does @app.get("/greet") def index(): """ TODO: 1. Capture first name & last name 2. If either is not provided: respond with an error 3. If first name is not provided and second name is provided: respond with "Hello Mr!" 4. If first name is provided byt second name is not provided: respond with "Hello, !" 5. If both names are provided: respond with a question, "Is your name """ return jsonify("TODO")
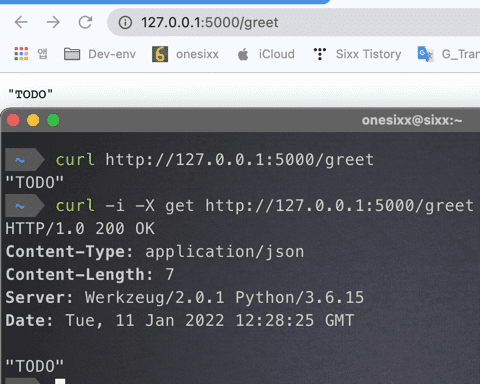
from flask import Flask, request, jsonify # Intitialise the app app = Flask(__name__) # Defiai axt the app does @app.get("/greet") def index(): fname = request.args.get("fname") lname = request.args.get("lname") if not fname and not lname: return jsonify({"status":"error"}) elif fname and not lname: response = {"data": f'Hello {fname} !!'} elif not fname and lname: response = {"data": f'Hello {lname} !!'} else: response = {"data":f"Hello {lname} {fname}!!"} return jsonify(response)
$ curl -i -X GET "http://127.0.0.1:5000/greet?fname=chung&lname=sixx"
p2-byog-flask : Building API Google search
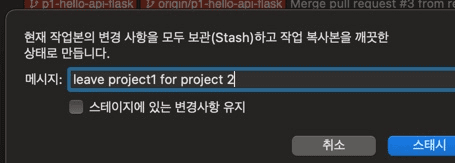
$ git stash $ git checkout p2-byog-flask $ conda install --file requirements.txt
working with URL kit
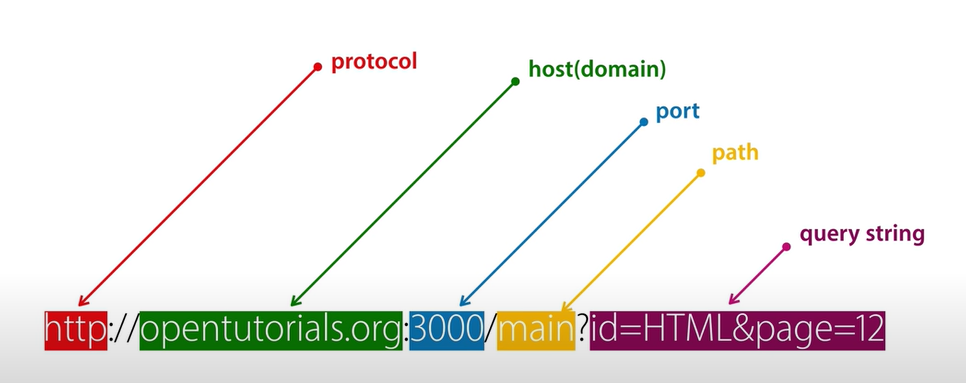
- templates : HTML pages
- static : template에서 사용하는 파일들 (이미지, javascript, css….)
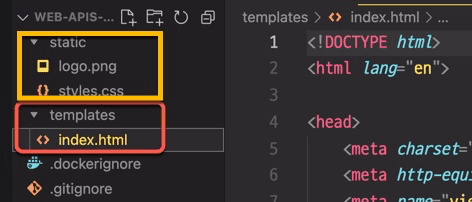
from flask import Flask, request, render_template, redirect app = Flask(__name__) @app.get("/") def index(): """ TODO: Render the home page provided under templates/index.html in the repository """ return render_template("index.html") @app.get("/search") def search(): """ TODO: 1. Capture the word that is being searched 2. Seach for the word on Google and display results """ args = request.args.get("q") return redirect(f"https://google.com/search?q={args}") if __name__ == "__main__": app.run()
p3-dictionary-api-flask : Creating dictionary API
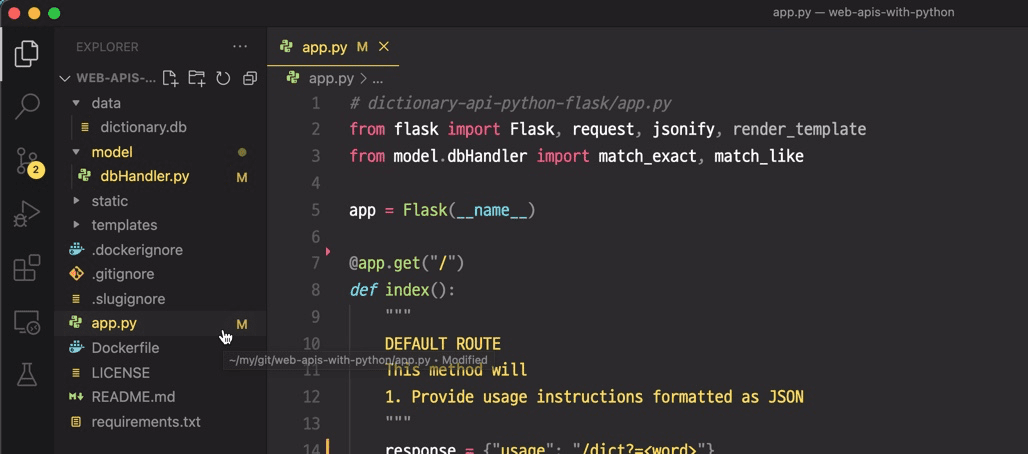
# dictionary-api-python-flask/app.py from flask import Flask, request, jsonify, render_template from model.dbHandler import match_exact, match_like app = Flask(__name__) @app.get("/") def index(): """ DEFAULT ROUTE This method will 1. Provide usage instructions formatted as JSON """ response = {"usage": "/dict?="} # Since this is a website with front-end, we don't need to send the usage instructions return jsonify(response) @app.get("/dict") def dictionary(): """ DEFAULT ROUTE This method will 1. Accept a word from the request 2. Try to find an exact match, and return it if found 3. If not found, find all approximate matches and return """ # word= request.args.get("word") words = request.args.getlist("word") if not words: response = {"status":"error", "data":"word not valid!", "word":words} return jsonify(response) response = {"words":[]} for word in words: definitions = match_exact(word) if definitions: response["words"].append({"status":"sccess", "data":definitions, "word":word}) print(f"111. {response}") else: definitions = match_like(word) if definitions: response["words"].append({"status":"partial", "data":definitions, "word":word}) else: response["words"].append({"status":"error", "data":"word not found", "word":word}) return jsonify(response) if __name__ == "__main__": app.run()
# dictionary-api-python-flask/model/dbHandler.py import sqlite3 as SQL def match_exact(word: str) -> list: db=SQL.connect("data/dictionary.db") sql_query="SELECT * FROM entries WHERE word=?" match = db.execute(sql_query, (word,)).fetchall() db.close() return match def match_like(word: str) -> list: db=SQL.connect("data/dictionary.db") sql_query="SELECT * FROM entries WHERE word LIKE ?" match = db.execute(sql_query, ("%"+word+"%",)).fetchall() db.close() return match
http://127.0.0.1:5000/dict?word=good
Template 과 static 사용
# dictionary-api-python-flask/app.py from flask import Flask, request, jsonify, render_template from model.dbHandler import match_exact, match_like app = Flask(__name__) @app.get("/") def index(): # response = {"usage": "/dict?="} # return jsonify(response) return render_template("index.html") @app.get("/dict") def dictionary(): words = request.args.getlist("word") if not words: response = {"status":"error", "data":"word not valid!", "word":words} return jsonify(response) #return render_template("results.html", response=jsonify(response)) response = {"words":[]} for word in words: definitions = match_exact(word) if definitions: response["words"].append({"status":"sccess", "data":definitions, "word":word}) print(f"111. {response}") else: definitions = match_like(word) if definitions: response["words"].append({"status":"partial", "data":definitions, "word":word}) else: response["words"].append({"status":"error", "data":"word not found", "word":word}) # return jsonify(response) return render_template("results.html", response=jsonify(response)) if __name__ == "__main__": app.run()
import requests URL="http://127.0.0.1:5000/dict?word=good" response=requests.get(URL) response.raise_for_status response.content
p4-image-filter-flask : Image Filter Adder API
filter /Route and Changes
https://pillow.readthedocs.io/en/stable/
from PIL import Image, ImageFilter import io def apply_filter(file: object, filter: str) -> object: """ TODO: 1. Accept the image as file object, and the filter type as string 2. Open the as an PIL Image object 3. Apply the filter 4. Convert the PIL Image object to file object 5. Return the file object """ image = Image.open(file) image = image.filter(eval( f"ImageFilter.{filter.upper()}")) file = io.BytesIO() image.save(file, "JPEG") file.seek(0) return file
from flask import Flask, request, send_file, jsonify from bin.filters import apply_filter app = Flask(__name__) # Read the PIL document to find out which filters are available out-of the box filters_available = [ "blur", "contour" ] @app.route("/", methods=["GET", "POST"]) def index(): """ TODO: 1. Return the usage instructions that specifies which filters are available, and the method format """ response ={ "filters_availble":filters_available, "usage":{"http_methods":"POST", "URL":"//"}, } return jsonify(response) @app.post("/ ") def image_filter(filter): """ TODO: 1. Checks if the provided filter is available, if not, return an error 2. Check if a file has been provided in the POST request, if not return an error 3. Apply the filter using apply_filter() method from bin.filters 4. Return the filtered image as response """ file = request.files["image"] filter_image = apply_filter(file, filter) return send_file(filter_image, mimetype="image/JPEG") if __name__ == "__main__": app.run()