Datatype (dict, list)
Number vs. String
Int, float, complex vs. 문자열
Built-in Collections
{set} vs. {: Dictionary}
Set | Dictionary |
key값만 있는 | key,value 값이 있다. |
가변(mutable) 리스트형 | – key값은 immutable(변경 불가)형식이어야 하고, – value값은 mutable(변경 가능) 형식도 가능하다 |
unordered collection of data | unordered collection of data that stores data in key-value pairs. |
(Tuple) vs. [List]
Tuple | List |
immutable | mutable |
수정이 불가능하다. an ordered collection of data. | 수정이 가능함 (ex. list.append() ) collection of ordered data. |
methods
#=== Using list slicing ### Extract sublists with list slicing numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] first_five = numbers[:5] last_five = numbers[5:] middle_five = numbers[3:8] evens = numbers[1::2] odds = numbers[::2] reversed_numbers = numbers[::-1] #=== Using string slicing ### Extract substrings with string slicing name = 'Alice' first_three = name[:3] last_three = name[2:] middle_three = name[1:4] evens = name[1::2] odds = name[::2] reversed_name = name[::-1] #=== Using list unpacking ### Unpack lists with list unpacking numbers = [1, 2, 3] a, b, c = numbers #=== Using dictionary unpacking ### Unpack dictionaries with dictionary unpacking person = {'name': 'Alice', 'age': 24} name, age = person.values() #=== Using set unpacking ### Unpack sets with set unpacking numbers = {1, 2, 3} a, b, c = numbers
#=== Using f-string ### Format strings with f-string name = 'Alice' age = 24 formatted_string = f'My name is {name} and I am {age} years old.' name = 'Alice' name_upper = name.upper() name_lower = name.lower() name_title = name.title() name_capitalize = name.capitalize() name_swapcase = name.swapcase() name_strip = name.strip() name_lstrip = name.lstrip() name_rstrip = name.rstrip() name_replace = name.replace('A', 'Z') name_split = name.split('l') name_join = '-'.join(['A', 'l', 'i', 'c', 'e'])
slicing
#=== Using sets to store unique values ### fileter distinct value, Set은 unordered key값만 있는 dictionary numbers = [2, 1, 1, 1, 2, 3, 3, 4, 5, 6, 7, 8, 5, 5] numbers = set(numbers) # {1, 2, 3, 4, 5, 6, 7, 8} june_dates = [datetime(2024, 6, day) for day in range(1, 31)] random_dates = random.sample(june_dates, k=16) # unordered dates, unique random_dates = random.choices(june_dates, k=16) # unordered dates, un-unique my_dates = set(random_dates) numbers.add(11) numbers.discard(5) numbers.remove(6) # 없는 값을 지우려고 하면 에러 발생 numbers.clear() #=== Using list methods ### Manipulate lists with list methods numbers = [1, 2, 3, 4, 5] numbers.append(6) numbers.insert(0, 0) numbers.extend([7, 8, 9]) numbers.pop(0) numbers.sort() numbers.remove(0) numbers.clear() #=== Using dictionary methods ### Manipulate dictionaries with dictionary methods person = {'name': 'Alice', 'age': 24} person['name'] = 'Bob' person['age'] = 50 person['city'] = 'New York' person.pop('city') person.popitem() person.clear()
Immutable vs. Mutable
변수가 담고 있는 object의 속성에 따라 결정
Immutable | mutable |
int, float, bool, string, tuple | list, dictionary |
json vs. dictionary
json
과 dictionary
모두 KEY
, VALUE
인데 왜 굳이 json
일까
Dictionary | JSON |
Data Structure | Data Format |
key는 어떤거든지… (key기본값 없음) | key는 항상 String (key기본값 undefined) |
True/ False / None | true/ false/ null |
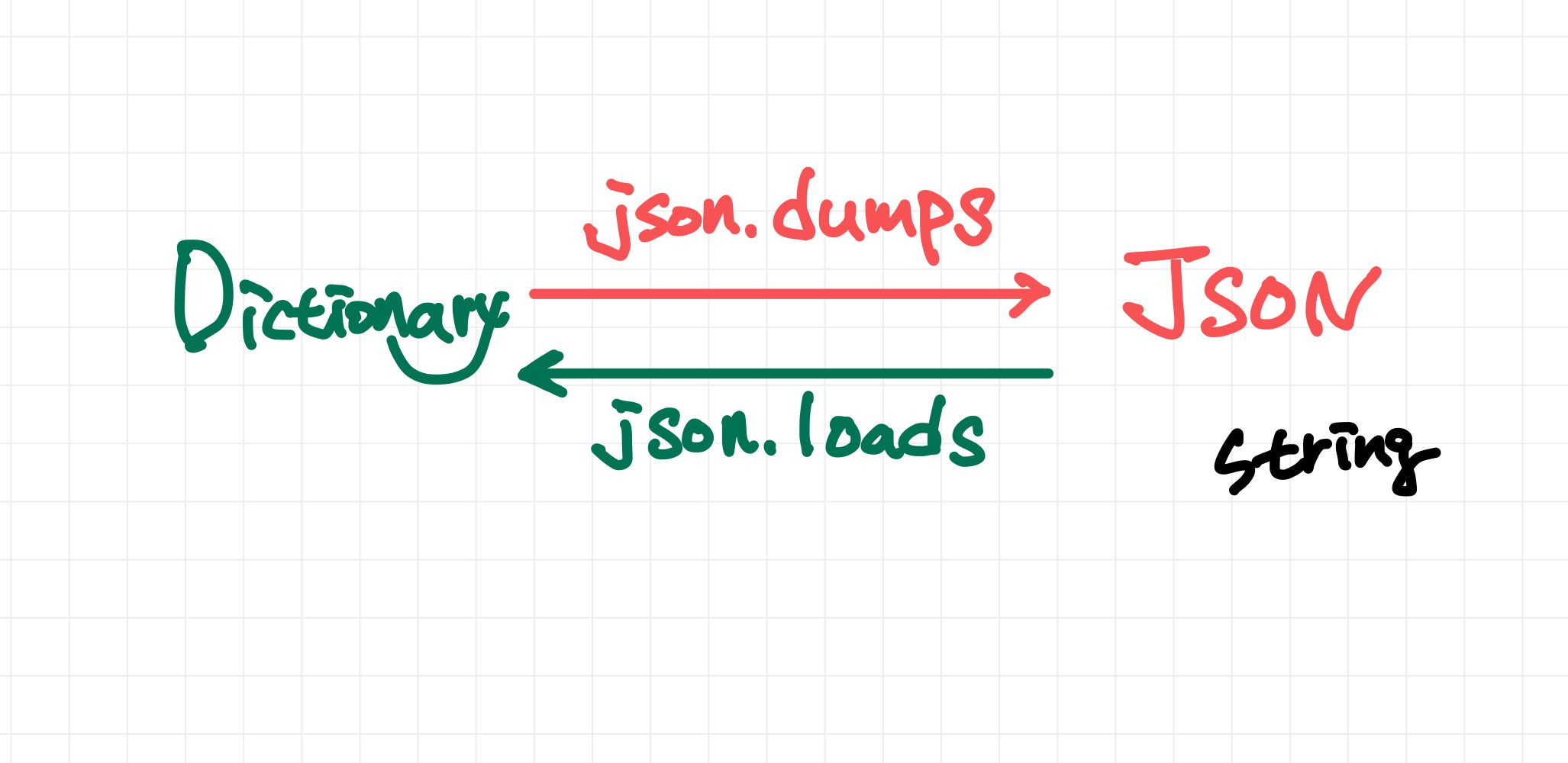
import json dictVal = {'name':'johnDou', 'age':16, 'job':True} jsonVal = json.dumps(dictVal) # '{"name": "johnDou", "age": 16, "job": true}' dictVal2 = json.loads(jsonVal) # {'name': 'johnDou', 'age': 16, 'job': True} dictVal['name'] # 'johnDou' jsonVal['name'] # TypeError: string indices must be integers