copilot
https://github.com/features/copilot
https://docs.github.com/en/copilot/quickstart
https://www.youtube.com/playlist?list=PLj6YeMhvp2S5_hvBl2SE-7YCHYlLQ0bPt
TAB
Ctrl + Enter
Cmd + [ ]
Cmd + ➡
q: a:
import datetime def parse_expenses(expenses_string): """Parse the list of expenses and return the list of triples (date, value, currency). Ignore lines starting with #. Parse the date using datetime. Example expenses_string: 2016-01-02 -34.01 USD 2016-01-03 2.59 DKK 2016-01-03 -2.72 EUR """ expenses = [] for line in expenses_string.splitlines(): if line.startswith("#"): continue date, value, currency = line.split(" ") expenses.append((datetime.datetime.strptime(date, "%Y-%m-%d"), float(value), currency)) return expenses
가입
https://docs.github.com/ko/copilot/getting-started-with-github-copilot?tool=visualstudio
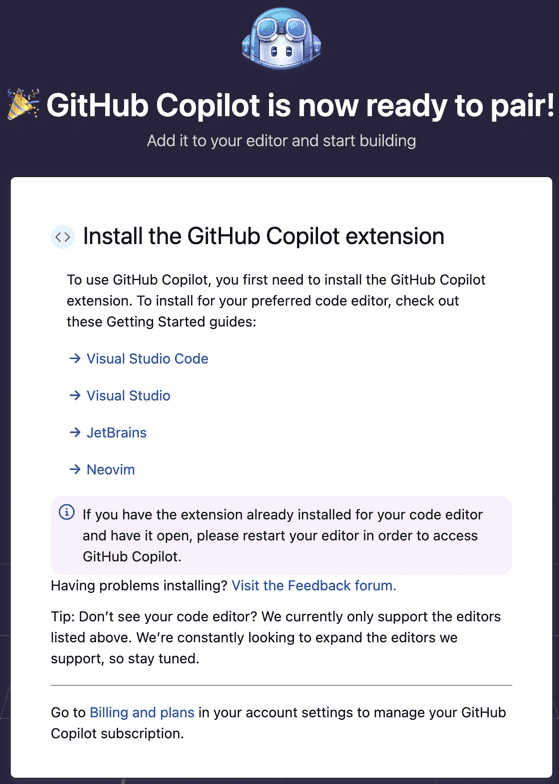
Extension설치
https://marketplace.visualstudio.com/items?itemName=GitHub.copilot
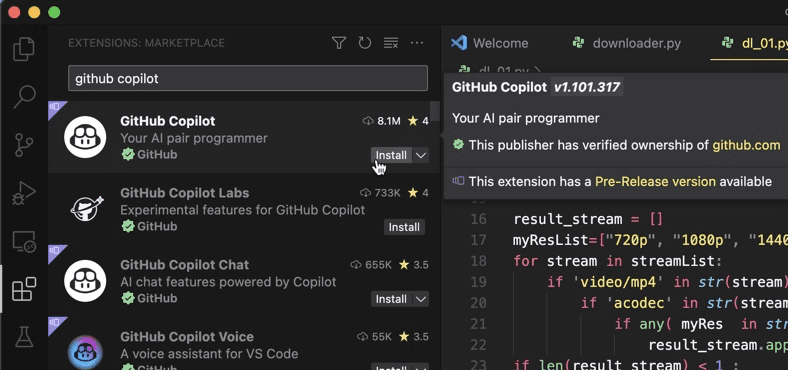
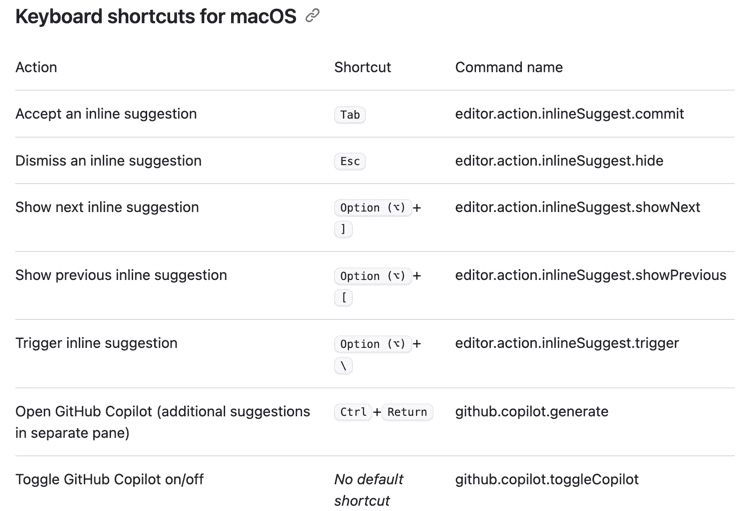
How to use GitHub Copilot: Prompts, tips, and use cases
https://github.blog/2023-06-20-how-to-write-better-prompts-for-github-copilot/
From documenting our codebases to generating unit tests, these tools are helping to accelerate our workflows.
However, just like with any emerging tech, there’s always a learning curve.
As a result, developers—beginners and experienced alike— sometimes feel frustrated when AI-powered coding assistants don’t generate the output they want. (Feel familiar?)
For example,
when asking GitHub Copilot to draw an ice cream cone ? using p5.js, a JavaScript library for creative coding, we kept receiving irrelevant suggestions—or sometimes no suggestions at all. But when we learned more about the way that GitHub Copilot processes information, we realized that we had to adjust the way we communicated with it.
Here’s an example of GitHub Copilot generating an irrelevant solution:
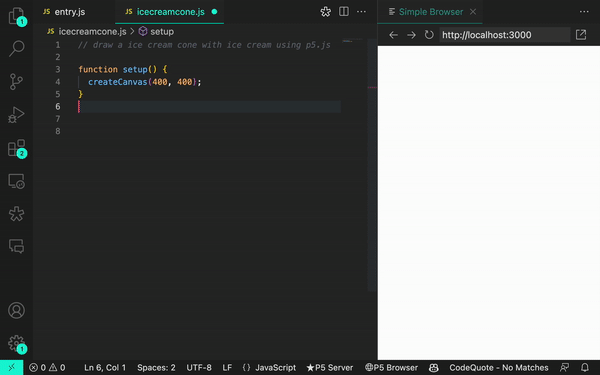
When we adjusted our prompt, we were able to generate more accurate results:
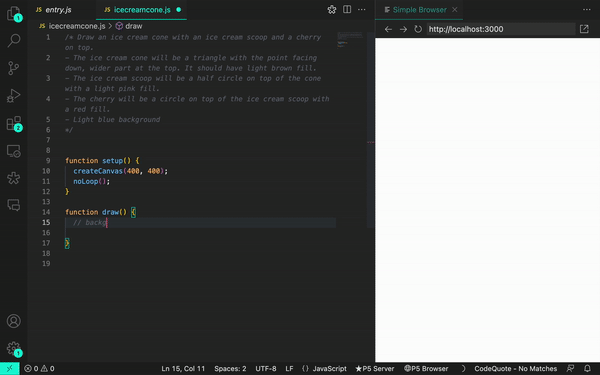
We’re both developers and AI enthusiasts ourselves. I, Rizel, have used GitHub Copilot to build a browser extension, rock, paper, scissors game, and to send a Tweet. And I, Michelle, launched an AI company in 2016. We’re both developer advocates at GitHub and love to share our top tips for working with GitHub Copilot.
In this guide for GitHub Copilot, we’ll cover:
- What exactly a prompt is and what prompt engineering is, too (hint: it depends on whether you’re talking to a developer or a machine learning researcher).
- Three best practices and three additional tips for prompt crafting with GitHub Copilot.
- An example where you can try your hand at prompting GitHub Copilot to assist you in building a browser extension.
Progress over perfection
Even with our experience using AI, we recognize that everyone is in a trial and error phase with generative AI technology. We also know the challenge of providing generalized prompt-crafting tips because models vary, as do the individual problems that developers are working on. This isn’t an end-all, be-all guide. Instead, we’re sharing what we’ve learned about prompt crafting to accelerate collective learning during this new age of software development.
What’s a prompt and what is prompt engineering?
It depends on who you talk to.
In the context of generative AI coding tools, a prompt can mean different things, depending on whether you’re asking machine learning (ML) researchers who are building and fine-tuning these tools, or developers who are using them in their IDEs.
For this guide, we’ll define the terms from the point of view of a developer who’s using a generative AI coding tool in the IDE. But to give you the full picture, we also added the ML researcher definitions below in our chart.
Prompts | Prompt engineering | Context | |
---|---|---|---|
Developer | Code blocks, individual lines of code, or natural language comments a developer writes to generate a specific suggestion from GitHub Copilot | Providing instructions or comments in the IDE to generate specific coding suggestions | Details that are provided by a developer to specify the desired output from a generative AI coding tool |
ML researcher | Compilation of IDE code and relevant context (IDE comments, code in open files, etc.) that is continuously generated by algorithms and sent to the model of a generative AI coding tool | Creating algorithms that will generate prompts (compilations of IDE code and context) for a large language model | Details (like data from your open files and code you’ve written before and after the cursor) that algorithms send to a large language model (LLM) as additional information about the code |
3 best practices for prompt crafting with GitHub Copilot
1. Set the stage with a high-level goal. ?️
This is most helpful if you have a blank file or empty codebase. In other words, if GitHub Copilot has zero context of what you want to build or accomplish, setting the stage for the AI pair programmer can be really useful. It helps to prime GitHub Copilot with a big picture description of what you want it to generate—before you jump in with the details.
When prompting GitHub Copilot, think of the process as having a conversation with someone: How should I break down the problem so we can solve it together? How would I approach pair programming with this person?
For example, when building a markdown editor in Next.jst, we could write a comment like this
/* Create a basic markdown editor in Next.js with the following features: - Use react hooks - Create state for markdown with default text "type markdown here" - A text area where users can write markdown - Show a live preview of the markdown text as I type - Support for basic markdown syntax like headers, bold, italics - Use React markdown npm package - The markdown text and resulting HTML should be saved in the component's state and updated in real time */
This will prompt GitHub Copilot to generate the following code and produce a very simple, unstyled but functional markdown editor in less than 30 seconds. We can use the remaining time to style the component:
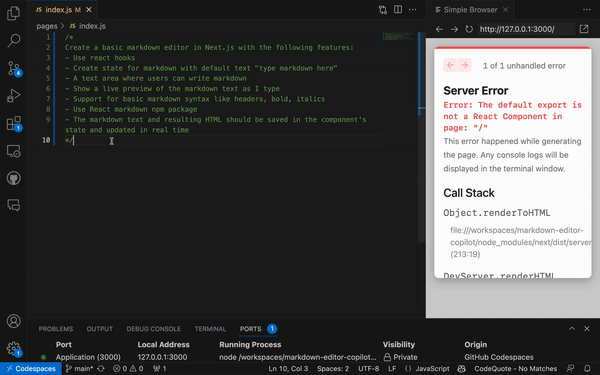
Note: this level of detail helps you to create a more desired output, but the results may still be non-deterministic. For example, in the comment, we prompted GitHub Copilot to create default text that says “type markdown here” but instead it generated “markdown preview” as the default words.
2. Make your ask simple and specific. Aim to receive a short output from GitHub Copilot. ?️
Once you communicate your main goal to the AI pair programmer, articulate the logic and steps it needs to follow for achieving that goal. GitHub Copilot better understands your goal when you break things down. (Imagine you’re writing a recipe. You’d break the cooking process down into discrete steps–not write a paragraph describing the dish you want to make.)
Let GitHub Copilot generate the code after each step, rather than asking it to generate a bunch of code all at once.
Here’s an example of us providing GitHub Copilot with step-by-step instructions for reversing a function:
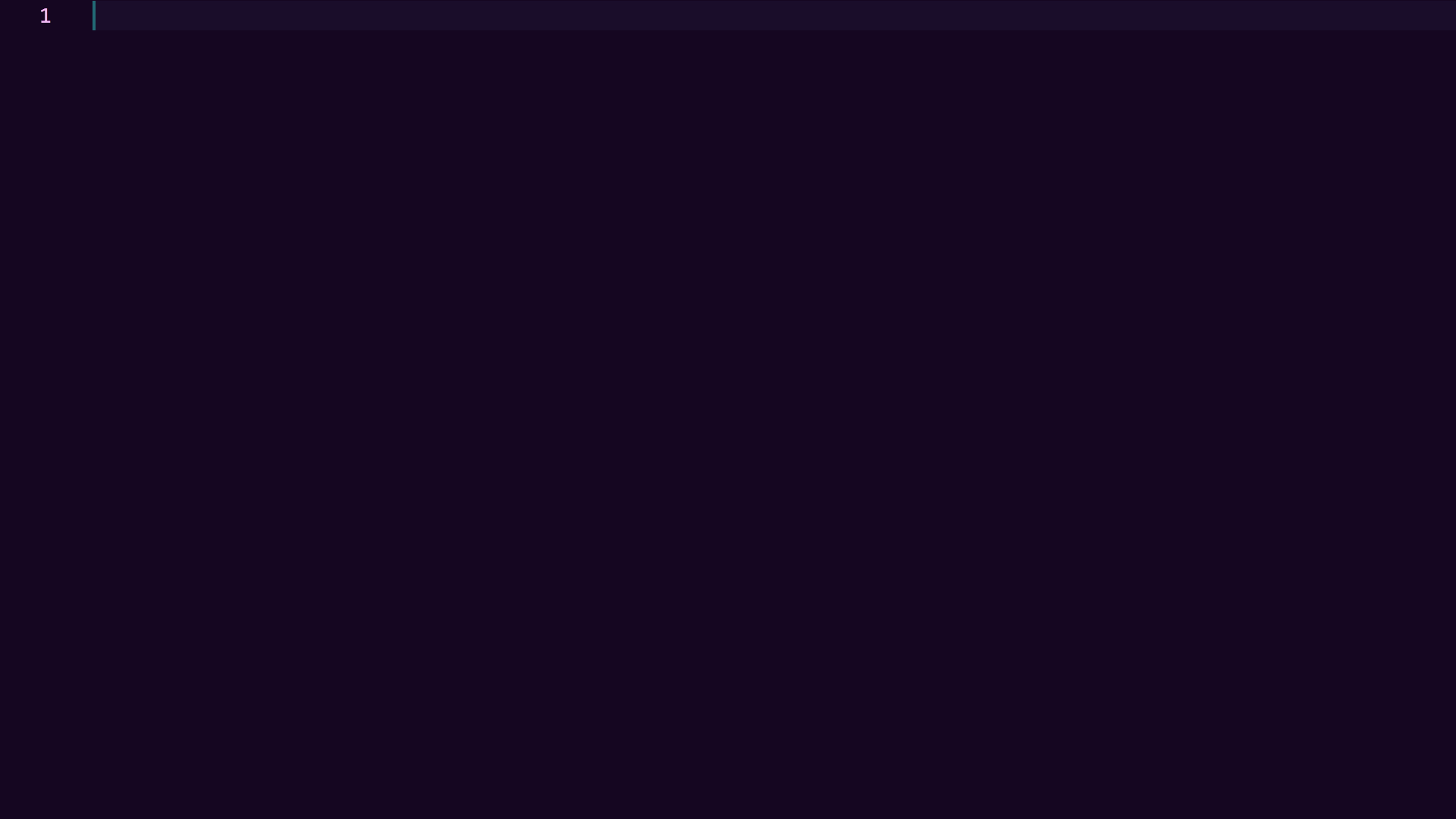
3. Give GitHub Copilot an example or two. ✍️
Learning from examples is not only useful for humans, but also for your AI pair programmer. For instance, we wanted to extract the names from the array of data below and store it in a new array:
const data = [ [ { name: 'John', age: 25 }, { name: 'Jane', age: 30 } ], [ { name: 'Bob', age: 40 } ] ];
When we didn’t show GitHub Copilot an example …
// Map through an array of arrays of objects to transform data const data = [ [ { name: 'John', age: 25 }, { name: 'Jane', age: 30 } ], [ { name: 'Bob', age: 40 } ] ]; const mappedData = data.map(x => [x.name](http://x.name/)); console.log(mappedData); // Results: [undefined, undefined]
It generated an incorrect usage of map:
const mappedData = data.map(x => [x.name](http://x.name/)); console.log(mappedData); // Results: [undefined, undefined]
By contrast, when we did provide an example …
// Map through an array of arrays of objects // Example: Extract names from the data array // Desired outcome: ['John', 'Jane', 'Bob'] const data = [ [{ name: 'John', age: 25 }, { name: 'Jane', age: 30 }], [{ name: 'Bob', age: 40 }] ]; const mappedData = data.flatMap(sublist => sublist.map(person => person.name)); console.log(mappedData);
We received our desired outcome.
const mappedData = data.flatMap(sublist => sublist.map(person => person.name)); console.log(mappedData); // Results: ['John', 'Jane', 'Bob']
Read more about common approaches to AI training, such as zero-shot, one-shot, and few-shot learning.
Three additional tips for prompt crafting with GitHub Copilot
Here are three additional tips to help guide your conversation with GitHub Copilot.
1. Experiment with your prompts.
Just how conversation is more of an art than a science, so is prompt crafting. So, if you don’t receive what you want on the first try, recraft your prompt by following the best practices above.
For example, the prompt below is vague. It doesn’t provide any context or boundaries for GitHub Copilot to generate relevant suggestions.
# Write some code for grades.py
We iterated on the prompt to be more specific, but we still didn’t get the exact result we were looking for. This is a good reminder that adding specificity to your prompt is harder than it sounds. It’s difficult to know, from the start, which details you should include about your goal to generate the most useful suggestions from GitHub Copilot. That’s why we encourage experimentation.
The version of the prompt below is more specific than the one above, but it doesn’t clearly define the input and output requirements.
# Implement a function in grades.py to calculate the average grade
We experimented with the prompt once more by setting boundaries and outlining what we wanted the function to do. We also rephrased the comment so the function was more clear (giving GitHub Copilot a clear intention to verify against).
This time, we got the results we were looking for.
# Implement the function calculate_average_grade in grades.py that takes a list of grades as input and returns the average grade as a floating-point number
2. Keep a couple of relevant tabs open.
We don’t have an exact number of tabs that you should keep open to help GitHub Copilot contextualize your code, but from our experience, we’ve found that one or two is helpful.
GitHub Copilot uses a technique called neighboring tabs that allows the AI pair programmer to contextualize your code by processing all of the files open in your IDE instead of just the single file you’re working on. However, it’s not guaranteed that GItHub Copilot will deem all open files as necessary context for your code.
3. Use good coding practices.
That includes providing descriptive variable names and functions, and following consistent coding styles and patterns. We’ve found that working with GitHub Copilot encourages us to follow good coding practices we’ve learned throughout our careers.
For example, here we used a descriptive function name and followed the codebase’s patterns of leveraging snake case.
def authenticate_user(username, password):
As a result, GitHub Copilot generated a relevant code suggestion:
def authenticate_user(username, password): # Code for authenticating the user if is_valid_user(username, password): generate_session_token(username) return True else: return False
Compare this to the example below, where we introduced an inconsistent coding style and poorly named our function.
def rndpwd(l):
Instead of suggesting code, GitHub Copilot generated a comment that said, “Code goes here.”
def rndpwd(l): # Code goes here
Stay smart
The LLMs behind generative AI coding tools are designed to find and extrapolate patterns from their training data, apply those patterns to existing language, and then produce code that follows those patterns. Given the sheer scale of these models, they might generate a code sequence that doesn’t even exist yet. Just as you would review a colleague’s code, you should always assess, analyze, and validate AI-generated code.
A practice example ???
Try your hand at prompting GitHub Copilot to build a browser extension.
To get started, you’ll need to have GitHub Copilot installed and open in your IDE. We also have access to an early preview of GitHub Copilot chat, which is what we’ve been using when we have questions about our code. If you don’t have GitHub Copilot chat, sign up for the waitlist. Until then you can pair GitHub Copilot with ChatGPT.
More generative AI prompt crafting guides
- A beginner’s guide to prompt engineering with GitHub Copilot
- Prompt engineering for AI
- How GitHub Copilot is getting better at understanding your code
Tags:
Error
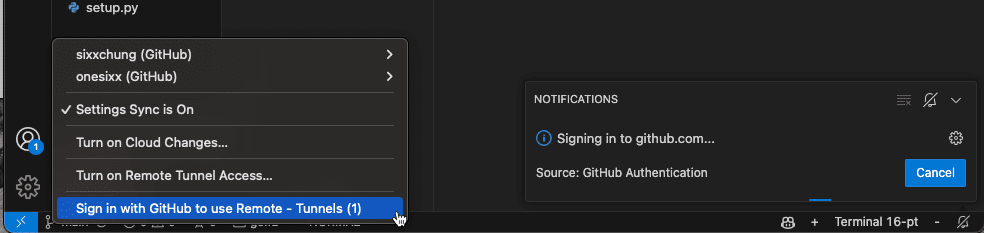