JSON
https://pynative.com/python-json-exercise/
Json 표준에는 겹따옴표가 표준이고 홑따옴표가 비표준이라는 것
vsCode에서 JSON파일
vscode ~ new file ~ select language : json
~ Shift+Option(alt)+ F ==> json 예쁘게 보기
dictionary -> json 문자열 :: json.dump()
json.dump() vs. json.dumps()
dump() 함수: Python객체를 JSON “파일”에 저장하기
dumps() 함수: Python객체를 JSON “문자열”로 변환
https://www.delftstack.com/ko/howto/python-pandas/json-to-pandas-dataframe/
더블쿼테이션이 표준
{ "1": "one", "2": "둘", "삼": "셋"}
{'1':'one', '2':'둘', '삼':'셋'}
import json # read : 파일 전체 # readline: 한라인데이터 # readlines: 전체데이터를 라인단위로 # data = '{"1":"one", "2":"둘", "삼":"셋"}' # str with open('../app/data/mobi-ex1.json', 'r') as line: data = line.read() s2d = json.loads(data) # {'1': 'one', '2': '둘', '삼': '셋'} # dict d2s = json.dumps(s2d) # '{"1": "one", "2": "\\\\ub458", "\\\\uc0bc": "\\\\uc14b"}' # str d2s_ko = json.dumps(s2d, ensure_ascii=False) # '{"1": "one", "2": "둘", "삼": "셋"}' # data = "{'1':'one', '2':'둘', '삼':'셋'}" # str with open('../app/data/mobi-ex1-single_quote.txt', 'r') as line: data = line.read() s2d = json.loads(data) # JSONDecodeError: Expecting property name enclosed in double quotes: line 1 column 2 (char 1) s2d = json.loads(data.replace("'", "\\""))
json문자열 -> dictionary :: json.load()
json.load() vs. json.loads()
load() 함수: JSON “파일”을 Python객체로 불러오기
loads() 함수: JSON “문자열”을 Python객체로 변환
json.load()
To parse JSON from URL or file
json.load( fp, *, cls=None, object_hook=None, parse_float=None, parse_int=None, parse_constant=None, object_pairs_hook=None, **kw)
fp: file pointer used to read a file,
json.loads()
For parse string with JSON content
into (python)Dictionary
json.loads( s, *, cls=None, object_hook=None, parse_float=None, parse_int=None, parse_constant=None, object_pairs_hook=None, **kw)
file pointer used to read a text file,
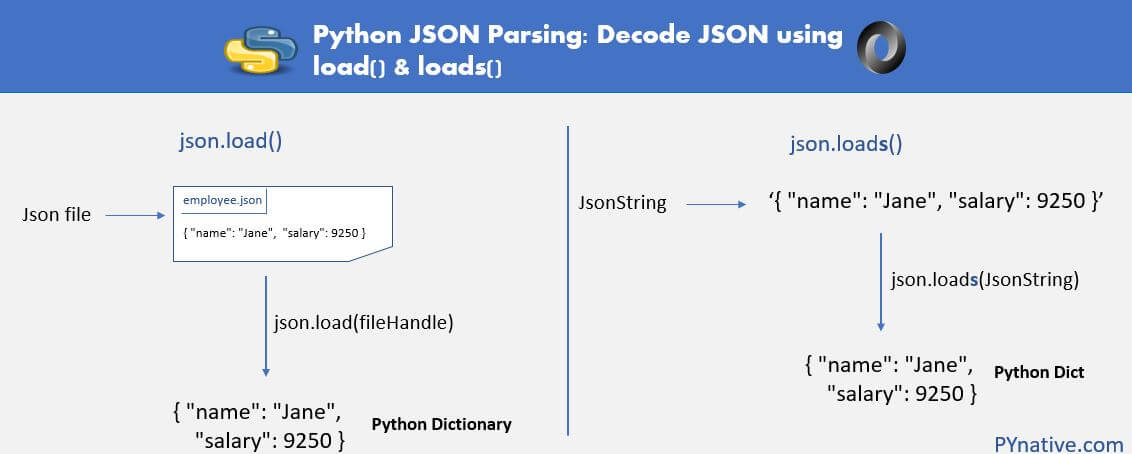
에러
AttributeError: ‘str’ object has no attribute ‘loads’
=> json.load()와 json.loads()를 구별못했거나, json이름을 변수로 사용한 경우
json 예제
{"id": 2, "name": ["def", "qwe"]} {"id": 3, "name": ["def", "asd"]} {"id": 4, "name": ["def", "zxc"]} ...
import json basePath = '../app/data/mobi_data' filePath = basePath + '/mobi1/mobility1_01.json' # json_data = open(filePath) # data = json.load(json_data) data = [] with open(filePath) as multiLines: for line in multiLines: data.append(json.loads(line))
read inline json
import json inline_JSON = '{"id":1, "name": ["abc", "xyz"]}' jsonData = json.loads(inline_JSON) #---------------------------------------------------------- len(jsonData) # 2 type(jsonData) # dict jsonData.keys() # dict_keys(['id', 'name']) jsonData.get("name") # ['abc', 'xyz'] json.dumps(jsonData) # '{"id": 1, "name": ["abc", "xyz"]}' print(json.dumps(jsonData, indent='\\t')) # { #\t"id": 1, #\t"name": [ #\t\t"abc", #\t\t"xyz" #\t] #}
read json Files
{ "id": 2, "name": ["def", "qwe"] }
path_JSON = './jsondata/jsn2.json' # String ### Read FilejsonFile = open(path_JSON,'r') #'r'ead,'w'rite,'a'ppend jsonData = json.load(jsonFile) jsonFile.close() ### Read File # with문을 나올 때 close를 자동으로 불러줍니다. with open(path_JSON) as jsonFile: jsonData = json.load(jsonFile)
JSON parse
### JSON (JavaScript Object Notation) # https://docs.python.org/3/library/json.html#json.dumps import json # Read string json_string='{"title": "example", "age": 38, "married": true}' contents = json.loads(json_string) # pretty print(json.dumps(contents, sort_keys=True ,indent=2)) # Load file with open('./text.json') as json_file: contents = json.load(json_file) print(json.dumps(contents, indent=4, ensure_ascii=False)) # Write JSON with open('./data.json', 'w') as json_file: json.dump(json_string, json_file) # parse 1 value val = contents["title"] val = contents.get("title") print(f'value=> {val}') # json to python # object to dictionaries # arrays to lists # Null to None valList = contents.get("house").get("json_array") for val in valList: print(val) valDic = contents.get("house").get("json_object") valDic.keys() valDic.values() for key in valDic: print(key, valDic[key]) for key, val in valDic.items(): print(key, val) for item in valDic.items(): print(item) # search with JMES Path import jmespath jmespath.search("persons[*].age", contents) jmespath.search("house.json_object.name", contents)
{ "title": "json_example", "house" : { "json_string": "string_example", "json_number": 100, "json_array": [1, 2, 3, 4, 5], "json_object": { "name":"John", "age":30}, "json_bool": true }, "house1" : { "json_string": "string_example", "json_number": 100, "json_array": [1, 2, 3, 4, 5], "json_object": { "name":"John", "age":30}, "json_bool": true }, "한글" : "그럴겁니다", "persons": [ { "name": "erik", "age": 38 }, { "name": "john", "age": 45 }, { "name": "rob", "age": 14 } ] }